Introduction
Frida is a powerful dynamic instrumentation toolkit that enables developers, security researchers, and enthusiasts to inspect and modify applications in real-time. While its primary use is in reverse engineering and security analysis, it’s also a handy tool for debugging and understanding complex apps. In this blog post, I will walk you through using Frida with an iOS app using an example project.
Prerequisites
Before diving in, ensure you have the following:
- A macOS environment with Xcode installed.
- An iOS device (jailbroken or non-jailbroken, depending on your use case).
- Frida installed on your system.
- Basic knowledge of iOS app development.
- Python (optional but helpful for scripting Frida).
Step 1: Setting Up Frida
- Install Frida on Your System
Frida can be installed using pip:
pip install frida-tools
- Check Frida Installation
Verify that Frida is installed correctly:
frida --version
This should return the version number.
- Install Frida on the iOS Device
For jailbroken devices, use the following command to install the Frida server:
ssh root@<device-ip> apt update apt install frida-server
Replace <device-ip>
with your device’s IP address.
Start the Frida server:
frida-server &
- Using Frida Without Jailbreak
If you are using a non-jailbroken device, you will need to leverage Frida’s gadget library. Here’s how you can do it:
- Download the Frida gadget library from the official Frida releases page.
- Embed the
frida-gadget.dylib
file into your app’s binary during development. - Modify your app’s
Info.plist
to load the Frida gadget:xml <key>LD_PRELOAD</key> <string>@executable_path/frida-gadget.dylib</string>
- Ensure proper code signing of the modified app before deploying it onto your device.
- Use Frida commands as usual to interact with the app. Note: This approach requires access to the app’s source code or a debuggable build.
Step 2: Create an Example iOS App
To demonstrate Frida’s capabilities, let’s create a simple iOS app in Xcode:
- Open Xcode and create a new project.
- Choose “App” and click “Next”.
- Provide a name (e.g.,
FridaDemoApp
), select Swift or Objective-C, and set the interface to “Storyboard”. - Add a basic UI with a button and a label.
- Implement a simple function triggered by the button to update the label’s text.
@IBAction func buttonTapped(_ sender: UIButton) { label.text = "Original Text Updated" }
Build and run the app on your device.
Step 3: Hook the App with Frida
- Find the App’s Process
Use Frida to list the processes running on your iOS device:
frida-ps -U
Identify your app’s process ID (PID).
- Attach to the App
Attach Frida to the app:
frida -U -n FridaDemoApp
- Write a Frida Script
Create a simple Frida script to modify the behavior of thebuttonTapped
function:
var hook = ObjC.classes.ViewController["- buttonTapped:"]; Interceptor.attach(hook.implementation, { onEnter: function (args) { console.log("buttonTapped function called!"); }, onLeave: function (retval) { console.log("Original return value: " + retval); retval.replace(ObjC.classes.NSString.stringWithString_("Modified by Frida")); } });
- Run the Script
Execute the script while the app is running:
frida -U -n FridaDemoApp -s script.js
Replace script.js
with the name of your script file.
- Observe Changes
Interact with the app’s button and observe how the behavior changes as per the Frida script.
Step 4: Automate with Python
For repetitive tasks, you can automate Frida scripting using Python:
import frida def on_message(message, data): print(message) session = frida.get_usb_device().attach("FridaDemoApp") script = session.create_script(open("script.js").read()) script.on('message', on_message) script.load() input("Press Enter to exit...")
Run the Python script:
python frida_automation.py
Best Practices and Considerations
- Always ensure you have permission to analyze or modify an app.
- Be mindful of legal and ethical considerations when using Frida.
- Use Frida for educational and security testing purposes.
Conclusion
Frida is a versatile tool for iOS app debugging, reverse engineering, and security analysis. With the example project above, you’ve seen how to hook into an iOS app and modify its behavior dynamically. This is just the beginning—Frida’s capabilities extend far beyond basic hooks, allowing you to explore and manipulate apps in depth.
Happy hacking!
Written By
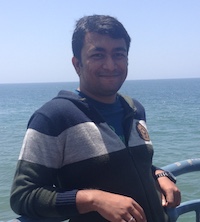
I’m working as an Enterprise Architect at Akamai Technologies. I have more than 14 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, Flutter, and other cross-platform apps. Have worked on 45+ apps from scratch, which are in the App Store and Play Store. My knowledge of mobile development includes design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities