Introduction
Man-in-the-Middle (MITM) attacks are a serious security threat for Android applications. They occur when an attacker secretly intercepts and manipulates communication between two parties—typically the user and the server. For Android developers, understanding how MITM attacks work and implementing measures to prevent them is critical to ensuring app security.
In this blog post, I’ll explore the basics of MITM attacks on Android apps and provide actionable steps to secure your applications against them.
What is a MITM Attack?
A MITM attack allows an attacker to intercept and potentially alter the data exchanged between a user and the app’s backend server. This could lead to stolen credentials, leaked sensitive information, or even unauthorized access to systems.
How Do MITM Attacks Work?
- Intercepting Traffic: An attacker intercepts network traffic using tools like a rogue Wi-Fi hotspot, ARP spoofing, or DNS spoofing.
- Decrypting Data: If traffic is not encrypted or uses weak encryption, the attacker can read sensitive data.
- Manipulating Data: The attacker modifies the data before sending it to the intended recipient, introducing vulnerabilities or executing malicious actions.
Common Tools for MITM Attacks
Attackers often use tools like:
- Burp Suite
- Charles Proxy
- MITMproxy
- Wireshark
These tools make it easy to intercept and inspect app traffic, especially if the app does not implement proper security practices.
How to Prevent MITM Attacks in Android Apps
1. Enforce HTTPS with SSL/TLS
- Always use HTTPS for all network communications.
- Ensure SSL/TLS certificates are valid and updated.
- Use services like Let’s Encrypt to obtain free SSL certificates.
2. Implement Certificate Pinning
Certificate pinning ensures that the app only accepts specific certificates or public keys, even if a valid but rogue certificate is presented.
Steps to Implement Certificate Pinning:
Example with OkHttp:
CertificatePinner certificatePinner = new CertificatePinner.Builder() .add("yourdomain.com", "sha256/AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=") .build(); OkHttpClient client = new OkHttpClient.Builder() .certificatePinner(certificatePinner) .build();
3. Use Network Security Configuration
Android provides the Network Security Configuration feature to customize app networking settings.
- Restrict unencrypted traffic by defining a
network_security_config.xml
:
<network-security-config> <domain-config cleartextTrafficPermitted="false"> <domain includeSubdomains="true">yourdomain.com</domain> </domain-config> </network-security-config>
Add the configuration in your AndroidManifest.xml
:
<application android:networkSecurityConfig="@xml/network_security_config" ... > </application>
4. Validate SSL Certificates
Always validate SSL certificates to ensure they are genuine. Use the HttpsURLConnection
or third-party libraries that handle proper certificate validation.
5. Monitor and Block Debugging Tools
- Detect rooted devices to reduce the risk of attackers using tools like Burp Suite or MITMproxy.
- Obfuscate app code using ProGuard or R8 to make reverse engineering difficult.
6. Educate Users
- Warn users about connecting to untrusted or open Wi-Fi networks.
- Encourage users to update apps and devices regularly for the latest security patches.
7. Use Custom Network Certificates and Validate Against Known Certificates
Implementing a custom network certificate allows your app to work only with trusted certificates. By validating the server’s network certificate against a list of known certificates, you can block malicious or rogue certificates. This step is particularly effective in preventing MITM attacks.
Steps to Use and Validate Custom Network Certificates:
- Generate a Custom Certificate: Create a custom certificate authority (CA) or obtain one from a trusted CA provider.
- Bundle Known Certificates in the App: Include the trusted server certificates in your app bundle.
- Validate Certificates Programmatically: When establishing a network connection, ensure that the server’s certificate matches one of the bundled known certificates.
Code Example for Validation:
Using X509TrustManager
for certificate validation:
TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm()); KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType()); InputStream certInputStream = context.getResources().openRawResource(R.raw.known_certificate); CertificateFactory certificateFactory = CertificateFactory.getInstance("X.509"); Certificate certificate = certificateFactory.generateCertificate(certInputStream); keyStore.load(null, null); keyStore.setCertificateEntry("server", certificate); trustManagerFactory.init(keyStore); SSLContext sslContext = SSLContext.getInstance("TLS"); sslContext.init(null, trustManagerFactory.getTrustManagers(), new SecureRandom()); OkHttpClient client = new OkHttpClient.Builder() .sslSocketFactory(sslContext.getSocketFactory(), (X509TrustManager) trustManagerFactory.getTrustManagers()[0]) .build();
Testing Your App’s Security
Before releasing your app, perform penetration testing using tools like:
- OWASP ZAP
- Burp Suite (in testing mode)
- SSL Labs (to validate SSL configurations)
Conclusion
MITM attacks are a persistent threat to Android applications. By using techniques like custom network certificates and validating them against known certificates, developers can add an extra layer of security. Combining this with HTTPS, certificate pinning, and proper SSL/TLS validation ensures robust protection against MITM threats.
Call to Action
Have more tips or questions about securing Android apps? Drop them in the comments below! Don’t forget to share this post with fellow developers to spread awareness about app security.
Written By
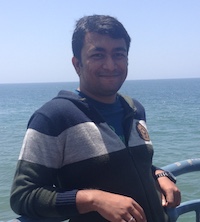
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 13 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities