Blockchain technology has revolutionized industries by introducing secure, decentralized systems. But have you ever wondered how a blockchain works under the hood? In this blog post, I’ll build a simple blockchain using Swift, step-by-step, to understand its core principles.
What is a Blockchain?
A blockchain is a chain of blocks, where each block contains data, a timestamp, and a hash that links it to the previous block. The hash ensures data integrity—if a block is tampered with, the hash changes, breaking the chain.
Setting Up the Project
Let’s dive into creating a simple blockchain in Swift.
1. Create a New Swift Project
- Open Xcode.
- Create a new macOS or iOS project.
- Choose a Command Line Tool template for simplicity.
- Name the project
Blockchain
.
Writing the Code
Step 1: Define the Block
Each block in the blockchain contains:
- Index
- Timestamp
- Data
- Previous Hash
- Current Hash
Here’s the code for a block:
import Foundation import CryptoKit // For hashing struct Block { let index: Int let timestamp: Date let data: String let previousHash: String var hash: String { let content = "\(index)\(timestamp)\(data)\(previousHash)" return SHA256.hash(data: content.data(using: .utf8)!) .map { String(format: "%02x", $0) } .joined() } }
Step 2: Define the Blockchain
The blockchain manages the list of blocks and ensures their integrity.
class Blockchain { private(set) var chain: [Block] = [] init() { // Create the genesis block addBlock(data: "Genesis Block") } func addBlock(data: String) { let previousHash = chain.last?.hash ?? "0000" let newBlock = Block( index: chain.count, timestamp: Date(), data: data, previousHash: previousHash ) chain.append(newBlock) } func isValid() -> Bool { for i in 1..<chain.count { let previousBlock = chain[i - 1] let currentBlock = chain[i] if currentBlock.previousHash != previousBlock.hash { return false } if currentBlock.hash != currentBlock.hash { return false } } return true } }
Step 3: Test the Blockchain
Now, let’s create and test the blockchain.
let blockchain = Blockchain() blockchain.addBlock(data: "Block 1 Data") blockchain.addBlock(data: "Block 2 Data") print("Blockchain:") for block in blockchain.chain { print(""" -------------------- Index: \(block.index) Timestamp: \(block.timestamp) Data: \(block.data) Previous Hash: \(block.previousHash) Hash: \(block.hash) """) } print("\nBlockchain Validity: \(blockchain.isValid() ? "Valid" : "Invalid")")
Output Example
When you run the code, you’ll see an output like this:
Blockchain: -------------------- Index: 0 Timestamp: 2024-11-22 06:52:46 +0000 Data: Genesis Block Previous Hash: 0000 Hash: 3333794d35a5edf9165c562cea2c883e8c94d46e93dcb6817a0d09e8b86706fe -------------------- Index: 1 Timestamp: 2024-11-22 06:52:46 +0000 Data: Block 1 Data Previous Hash: 3333794d35a5edf9165c562cea2c883e8c94d46e93dcb6817a0d09e8b86706fe Hash: 20962ef4d47b5f09b39c576a8b658a90318c3761c8dae7b8f227947475043ad6 -------------------- Index: 2 Timestamp: 2024-11-22 06:52:46 +0000 Data: Block 2 Data Previous Hash: 20962ef4d47b5f09b39c576a8b658a90318c3761c8dae7b8f227947475043ad6 Hash: f118ae5c5a0679b513790847d015cd1b1a9c47599101c99bd983adaa0365d92a Blockchain Validity: Valid
What’s Next?
Now that you’ve built a simple blockchain, you can explore advanced features like:
- Proof of Work: Introduce mining and difficulty levels.
- Decentralization: Simulate a peer-to-peer network.
- Transactions: Add transaction structures for practical use cases.
Conclusion
Building a blockchain in Swift is an excellent way to understand how this technology works. While my example is basic, it lays the foundation for creating more complex systems. Try it out, and let me know your thoughts in the comments below! Also, I have added the complete project for your reference.
https://github.com/fareethjohn/SwiftBlockchain
Happy coding! 🚀
Written By
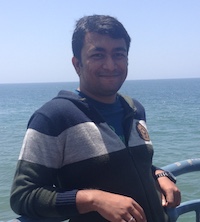
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 13 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities