Gradle is the backbone of Android app development in Android Studio. It automates and manages the build process, making it possible to efficiently compile, test, and package your applications. For developers, a strong understanding of Gradle and its configuration can significantly streamline the development workflow and avoid potential pitfalls.
What is Gradle and Its Role in Android Development?
Gradle is a build automation tool used in Android Studio for managing project dependencies, compiling code, running tests, and generating APKs or AABs for release. It uses a Groovy or Kotlin DSL (Domain-Specific Language) for configuration.
Key Roles of Gradle in Android Development
- Dependency Management: Fetch and integrate libraries from repositories like Maven or JCenter.
- Build Variants: Handle multiple versions of an app (e.g.,
debug
andrelease
). - Task Automation: Automate repetitive tasks like resource shrinking, obfuscation, and signing.
- Custom Build Logic: Tailor build configurations for specific needs.
Key Properties and Configurations in Gradle
Gradle configuration in Android is defined in two main files:
- Project-level
build.gradle
: Manages configurations for all modules. - Module-level
build.gradle
: Contains configurations specific to the app module.
Important Properties and Configurations
1. Project-Level Build Script
- Repositories: Define where dependencies are fetched from (e.g.,
google()
,mavenCentral()
). - Dependencies: Include build tools like
com.android.tools.build:gradle
.
Example:
groovyCopy codebuildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath "com.android.tools.build:gradle:8.1.0"
}
}
2. Module-Level Build Script
- Compile SDK Version: Specifies the Android SDK version used for compiling.
- Default Config: Defines application ID, minimum and target SDK versions, and versioning.
- Build Types: Specifies settings for
debug
andrelease
builds. - Dependencies: Includes libraries required for the app.
Example:
groovyCopy codeandroid {
compileSdkVersion 34
defaultConfig {
applicationId "com.example.myapp"
minSdkVersion 21
targetSdkVersion 34
versionCode 1
versionName "1.0"
}
buildTypes {
debug {
debuggable true
}
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation "androidx.appcompat:appcompat:1.6.1"
implementation "com.google.android.material:material:1.9.0"
}
3. Gradle Properties
- Defined in the
gradle.properties
file to improve build performance or set global properties. - Example:propertiesCopy code
org.gradle.daemon=true org.gradle.parallel=true android.useAndroidX=true
Common Mistakes in Gradle Configurations and How to Fix Them
1. Incorrect Dependency Versions
Mistake: Adding conflicting versions of a library.
Fix: Use the dependency resolution strategy to resolve conflicts.
groovyCopy codeconfigurations.all {
resolutionStrategy {
force 'com.example.library:1.2.3'
}
}
2. Slow Build Times
Mistake: Not enabling Gradle caching or parallel builds.
Fix: Optimize gradle.properties
:
propertiesCopy codeorg.gradle.caching=true
org.gradle.parallel=true
3. Version Compatibility Issues
Mistake: Using incompatible Gradle plugin and Android Studio versions.
Fix: Always refer to the Android Gradle plugin version compatibility table and update as needed.
4. Missing ProGuard Rules
Mistake: App crashes in release
builds due to aggressive code shrinking.
Fix: Add appropriate ProGuard rules in proguard-rules.pro
:
proCopy code-keep class com.example.** { *; }
5. Outdated SDK or Tools
Mistake: Using an outdated compileSdkVersion
or dependencies.
Fix: Regularly update SDK versions and libraries to the latest stable release.
6. Hardcoding Credentials
Mistake: Including sensitive information in Gradle files.
Fix: Use environment variables or Gradle properties:
groovyCopy codebuildTypes {
release {
buildConfigField("String", "API_KEY", "\"${System.getenv('API_KEY')}\"")
}
}
7. ABI Compatibility Issues with abiFilters
Mistake:
Using abiFilters
to restrict the app to specific CPU architectures, but unintentionally excluding required ABIs for third-party libraries. This can cause crashes on devices that rely on missing ABIs.
Why It Happens:
- When
abiFilters
is configured, Gradle includes only the specified ABIs during the build process. - If a third-party library relies on native code for an ABI not included in the filters, the app will crash at runtime with errors like
java.lang.UnsatisfiedLinkError
.
Tips for Managing Gradle Effectively
- Modularize Projects: Divide your app into smaller modules to reduce build time.
- Use Dependency Constraints: Prevent unexpected upgrades.
- Enable Build Analyzer: Use Android Studio’s Build Analyzer tool to identify bottlenecks.
- Regular Cleanup: Clear Gradle cache periodically with
gradlew clean
.
Conclusion
Gradle is a powerful tool that provides flexibility and efficiency in Android app development. By understanding its configurations and avoiding common pitfalls, developers can significantly improve build performance and maintain a robust project structure. Dive deeper into Gradle’s documentation and features to master its capabilities for your next Android project.
Happy coding! 🚀
Written By
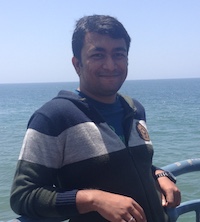
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 13 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities