Smali code provides a way to dive deep into the inner workings of Android apps, offering developers and enthusiasts the ability to implement custom features. By editing the app’s Smali code, you can enhance functionality, add new behaviors, or tweak existing features. This guide will walk you through the process of implementing custom features in Android apps using Smali.
What is Smali?
Smali is the assembly language for the Dalvik Virtual Machine (DVM) used in Android apps. It represents the bytecode in a human-readable format, making it possible to modify an app’s logic and behavior at a low level.
By editing Smali code, you can:
- Add new methods or features to an app.
- Customize UI elements or logic.
- Integrate logging or debugging mechanisms.
- Modify app workflows to suit specific needs.
Prerequisites
- Basic understanding of Smali syntax.
- APKTool: To decompile and recompile APK files.
- Java Development Kit (JDK): Required for APKTool and APK signing.
- Text Editor: VS Code or Notepad++ with Smali extensions.
- Android Emulator or Device for testing.
Step 1: Decompile the APK
To begin, decompile the APK using APKTool to access the app’s Smali code.
apktool d app.apk
This creates a folder containing the Smali files in the smali/
directory.
Step 2: Identify the Target Class
Determine the class or method where you want to add your custom feature. This can be done by examining the app’s flow or using tools like JADX to inspect the app’s structure.
Step 3: Add a Custom Method
To implement a custom feature, you’ll typically need to add a new method to a relevant class. Here’s an example of adding a logging method.
Example: Adding a Logging Method
Insert the following Smali code into the target class:
.method public logCustomMessage()V
.locals 1
const-string v0, "Custom Feature Triggered!"
invoke-static {v0}, Landroid/util/Log;->d(Ljava/lang/String;Ljava/lang/String;)I
return-void
.end method
This method logs a custom message to the Logcat when triggered.
Step 4: Call the Custom Method
Next, find a suitable location in the app’s existing code where you can invoke the custom method. For instance, you can add the following line to an existing method:
invoke-direct {p0}, Lcom/example/MyClass;->logCustomMessage()V
This ensures the custom method is called when the target method is executed.
Step 5: Recompile the APK
After making the necessary modifications, recompile the APK using APKTool:
apktool b app_folder
The recompiled APK will be located in the dist/
folder.
Step 6: Sign the APK
Android requires signed APKs for installation. Use jarsigner
or another tool to sign the APK:
jarsigner -verbose -keystore my-release-key.keystore app.apk alias_name
Step 7: Install and Test
Install the modified APK on an Android device or emulator:
adb install app.apk
Run the app and verify that your custom feature is functioning as intended. For example, check the Logcat output to see your custom log message.
Best Practices
- Backup: Always back up the original APK and Smali files before making changes.
- Test Thoroughly: Test your custom feature in various scenarios to ensure it works correctly.
- Respect Legal Boundaries: Only modify apps you own or have permission to modify.
- Use Meaningful Logs: Add meaningful log messages for easier debugging.
Conclusion
Implementing custom features in Android apps using Smali can be a rewarding challenge. By following these steps, you can enhance app functionality, add unique behaviors, and gain deeper insights into Android development. Remember to use your skills responsibly and ethically.
Start experimenting today and unleash the full potential of Smali code!
Written By
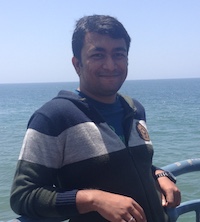
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 12 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities