Smali code allows you to modify Android apps at the bytecode level, giving you precise control over their behavior. Whether you want to tweak app logic, remove restrictions, or simply experiment, Smali offers a unique way to achieve these goals. In this guide, I’ll explain how to modify an app’s behavior by editing Smali code, step-by-step.
Prerequisites
- Basic understanding of Android app structure and Smali syntax.
- APKTool: To decompile and recompile APK files.
- Java Development Kit (JDK): Required for APKTool and signing APKs.
- Text Editor: VS Code or Notepad++ with Smali syntax highlighting.
- Android Emulator or Device: For testing your changes.
Step 1: Decompile the APK
The first step in modifying an app’s behavior is to decompile the APK file to access its Smali code.
apktool d app.apk
This command generates a folder containing the app’s Smali code and resources. Look for the smali/
directory, where the app’s logic is stored in Smali files.
Step 2: Locate the Method or Class to Modify
Find the class or method responsible for the behavior you want to modify. This can be done by:
- Examining log messages or error codes in the app.
- Using a decompiler like JADX to inspect the app’s Java code for hints.
- Searching for keywords in the Smali files.
For example, if you want to change a button’s action, locate the onClick
method for the button’s ID in the Smali code.
Step 3: Modify the Smali Code
Once you’ve found the relevant method, you can edit its behavior by modifying the Smali instructions. Let’s look at an example where we alter a method that checks for premium access.
Original Smali Code
.method public isPremium()Z
.locals 1
const/4 v0, 0x0 # false
return v0
.end method
Modified Smali Code
Change the return value from false
to true
:
.method public isPremium()Z
.locals 1
const/4 v0, 0x1 # true
return v0
.end method
Save your changes after editing.
Step 4: Recompile the APK
After making the necessary changes, recompile the APK using APKTool:
apktool b app_folder
The recompiled APK will be found in the dist/
folder.
Step 5: Sign the Modified APK
Android requires APKs to be signed before installation. Use jarsigner
or another signing tool to sign the APK:
jarsigner -verbose -keystore my-release-key.keystore app.apk alias_name
Ensure the keystore is properly configured before running this command.
Step 6: Install and Test
Install the modified APK on an emulator or device to verify that the behavior has been successfully changed:
adb install app.apk
Launch the app and test the modified feature. For example, if you edited the isPremium
method, check whether the app behaves as if premium access is enabled.
Best Practices
- Backup Files: Always keep a backup of the original APK and Smali files before making changes.
- Analyze Carefully: Understand the code logic before modifying it to avoid breaking functionality.
- Test Thoroughly: Ensure the changes work as expected and don’t cause unexpected issues.
- Respect Copyright Laws: Only modify apps you own or have permission to modify.
Conclusion
Editing Smali code to modify an app’s behavior is a powerful skill that opens up endless possibilities. With practice and the right tools, you can tweak app functionality, debug issues, and learn more about Android internals. Always remember to use your skills ethically and within the bounds of the law.
Start experimenting today and unlock the potential of Smali code!
Written By
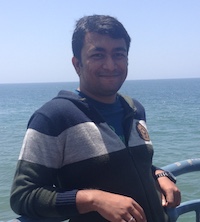
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 13 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities