In this case study, I will explore how to remove a login screen from an Android app by editing its Smali code. This exercise demonstrates the power of Smali code for modifying app behavior. For educational purposes only, this guide assumes you have the necessary permissions to modify the app.
What is Smali Code?
Smali is the human-readable assembly language for the Dalvik Virtual Machine (DVM) used in Android apps. By decompiling an APK file, you can view and edit its Smali code, which allows you to customize app behavior, including bypassing or removing features like login screens.
Prerequisites
- APKTool: For decompiling and recompiling APKs.
- Java Development Kit (JDK): Required for APKTool and APK signing.
- Text Editor: VS Code or Notepad++ with Smali syntax highlighting.
- An Android device or emulator for testing.
Step 1: Decompile the APK
First, use APKTool to decompile the APK file and access its Smali code:
apktool d app.apk
This command creates a folder with the app’s resources and Smali files in the smali/
directory.
Step 2: Identify the Login Logic
To remove the login screen, identify the Smali file or method responsible for the login logic. Common approaches include:
- Searching for keywords like
login
,auth
, orauthentication
in the Smali files. - Using JADX to inspect the app’s Java code for methods related to login.
- Locating UI elements in
res/layout/
that correspond to the login screen.
Step 3: Edit the Smali Code
Once you locate the method controlling the login logic, edit the Smali code to bypass it. For example, if the method requires a boolean value for login success, you can modify the code to always return true
.
Original Smali Code
.method public isAuthenticated()Z .locals 1 invoke-static {}, Lcom/example/AuthManager;->checkLogin()Z move-result v0 return v0 .end method
Modified Smali Code
Change the method to always return true
:
.method public isAuthenticated()Z .locals 1 const/4 v0, 0x1 # true return v0 .end method
Step 4: Recompile the APK
Recompile the APK using APKTool:
apktool b app_folder
The recompiled APK will be available in the dist/
folder.
Step 5: Sign the APK
Use jarsigner
or another signing tool to sign the modified APK:
jarsigner -verbose -keystore my-release-key.keystore app.apk alias_name
Step 6: Install and Test
Install the signed APK on an Android device or emulator:
adb install app.apk
Launch the app and verify that the login screen has been bypassed. Test the app thoroughly to ensure no additional functionality is affected.
Best Practices
- Backup: Always back up the original APK and extracted files before editing.
- Understand the Logic: Analyze the code thoroughly to avoid breaking other features.
- Respect Permissions: Only modify apps you own or have explicit permission to alter.
- Test Extensively: Test the modified app in different scenarios to ensure stability.
Conclusion
Removing a login screen using Smali code showcases the flexibility and power of reverse engineering Android apps. While this case study serves as an educational example, always respect ethical guidelines and legal boundaries when working with apps. With practice, Smali code can unlock a deeper understanding of Android app behavior and functionality.
Start experimenting today and unleash the full potential of Smali code!
Written By
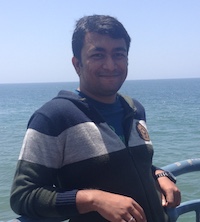
I’m working as a Sr. Solution Architect in Akamai Technologies. I have more than 13 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, flutter, and other cross-platform apps. Have worked on 45+ apps from scratch which are in the AppStore and PlayStore. My knowledge of mobile development including design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities