Frida is a dynamic instrumentation toolkit widely used for debugging, reverse engineering, and application security testing. In this blog post, I’ll explore how to use Frida in an Android app running on a simulator. I’ll also provide a complete example project to help you get started quickly.
What is Frida?
Frida is a powerful tool that allows you to inject scripts into native apps for introspection, debugging, or modification. It is widely used in penetration testing and reverse engineering for tasks like API monitoring, bypassing security checks, or inspecting app behavior.
Why Use Frida on a Simulator?
Using a simulator has several advantages:
- No physical device is required.
- Easier setup and debugging.
- Repeatable and isolated environment for testing.
Prerequisites
Before you start, ensure the following:
- Simulator/Emulator: An Android emulator (e.g., Android Studio AVD or Genymotion).
- Frida Installed: Install Frida on your system by running:
pip install frida-tools
- Rooted Emulator: Root access on the emulator is required for many Frida operations.
Setting Up Frida on an Android Emulator
Step 1: Start the Emulator
Launch your emulator from Android Studio or your preferred virtualization platform.
Step 2: Install the Frida Server on the Emulator
- Download Frida Server:
- Visit the Frida releases page.
- Download the appropriate Frida server version for your emulator’s architecture (e.g., x86 for Android Studio AVD).
- Push the Frida Server to the Emulator: Transfer the server binary to the emulator using
adb
:adb push frida-server /data/local/tmp/
- Set Permissions: Make the Frida server executable:
adb shell chmod +x /data/local/tmp/frida-server
- Run the Frida Server: Start the server on the emulator:
adb shell ./data/local/tmp/frida-server &
Step 3: Connect to the Emulator
Check the emulator’s IP address (default is 127.0.0.1
) and forward the port:
adb forward tcp:27042 tcp:27042
Example Project: Hooking a Method in a Sample App
Let’s demonstrate how to hook into a method in a sample app.
Step 1: Create a Sample Android App
Build a simple Android app with a method to hook. For example:
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Log.d("TestApp", "Original Method Called"); } }
Step 2: Write a Frida Script
Create a script to hook the onCreate
method:
Java.perform(function () { var MainActivity = Java.use("com.example.testapp.MainActivity"); MainActivity.onCreate.implementation = function (bundle) { console.log("Hooked Method Called"); this.onCreate(bundle); // Call original method }; });
Save this script as hook.js
.
Step 3: Run the Script
- Start the Frida CLI and attach to the app:
frida -U -n com.example.testapp
- Load the script:
% load hook.js
- Monitor the output in the terminal.
Verifying the Hook
- Launch the app in the emulator.
- Check the terminal for the message
Hooked Method Called
. - Confirm that the app’s original functionality remains unaffected.
Common Issues and Solutions
1. Frida Server Not Running
- Ensure you have pushed the correct Frida server binary.
- Verify the emulator is rooted.
2. App Crashes
- Check for anti-debugging mechanisms in the app. Use Frida scripts to bypass them.
3. Connection Errors
- Verify port forwarding with
adb forward
. - Ensure Frida server is running on the correct port.
Conclusion
Frida is a versatile tool that can help you explore and debug Android apps effectively. Running it on a simulator provides a controlled environment for safe experimentation. With the steps and example provided, you should be able to start using Frida in your Android app development or testing workflow.
Happy debugging! If you have any questions or run into issues, feel free to leave a comment below.
Written By
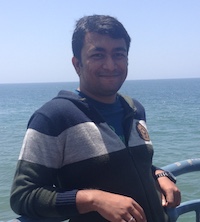
I’m working as an Enterprise Architect at Akamai Technologies. I have more than 14 years of experience in the Mobile app development industry. Worked on different technologies like VR, Augmented reality, OTT, and IoT in iOS, Android, Flutter, and other cross-platform apps. Have worked on 45+ apps from scratch, which are in the App Store and Play Store. My knowledge of mobile development includes design/architecting solutions, app development, knowledge around backend systems, cloud computing, CDN, Test Automation, CI/CD, Frida Pentesting, and finding mobile app vulnerabilities